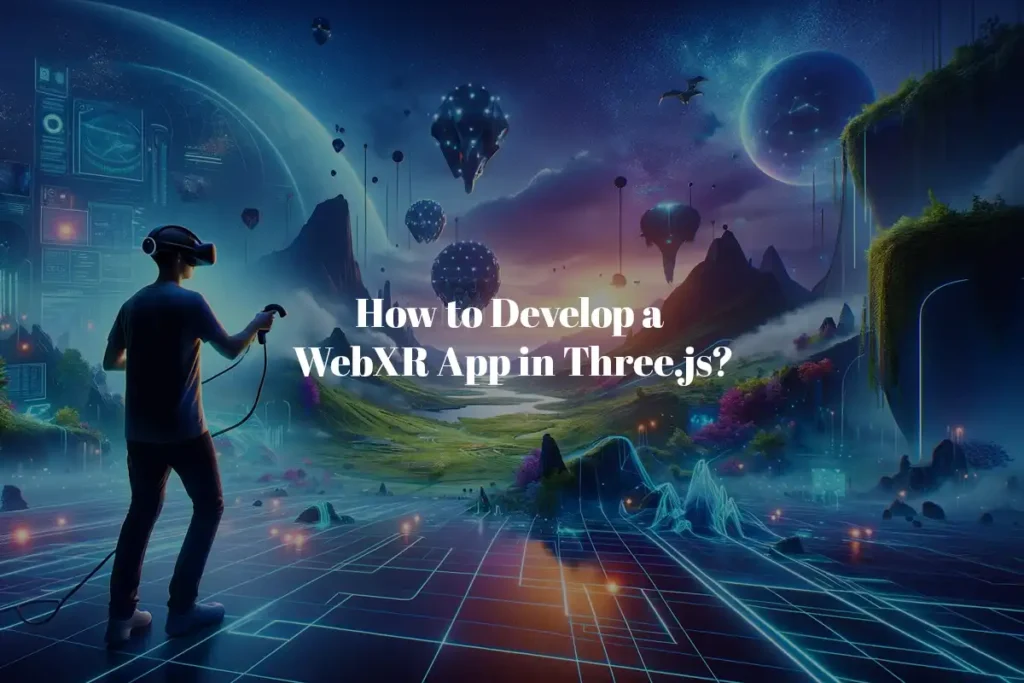
WebXR is an emerging web standard providing access to augmented reality (AR) and virtual reality (VR) technology to the browser. Using WebXR, developers can create impressive 3D apps that work across devices without needing to install native apps or plugins.
In this guide, we will walk through the end-to-end process of building WebXR apps with Three.js – the most popular open-source library for crafting 3D graphics on the web. In the end, you will have the skills to create interactive 3D worlds that users can experience right in their browsers.
What is WebXR?
WebXR refers to a set of APIs and best practices that aim to make immersive 3D apps accessible on the open web platform. The WebXR Device API allows you to interface with AR/VR hardware to render stereoscopic views and track user movement. WebXR also handles presenting these views properly on the device display.
Some key capabilities offered by WebXR include:
- Stereoscopic rendering to VR/AR headsets
- Tracking the position and orientation of VR headsets/AR mobile devices
- Processing user input from motion controllers
- Displaying consistent views for each eye on VR headsets
Using WebXR with a 3D library like Three.js, you can start building highly interactive 3D apps for the web – no native development required!
Step-by-Step WebXR App Development Guide
1. Setting Up Your Development Environment
To build WebXR experiences, the first step is making sure your dev environment has all the necessary tools and is properly configured.
Installing Core Tools and Dependencies
- Node.js: Install the latest LTS release of Node.js for access to npm
- npm: Package manager for installing JavaScript libraries like Three.js
- Three.js: 3D graphics library to create animated 3D scenes
Setting Up a Local Development Server
- Use webpack to bundle your Three.js code and assets
- Set up a dev server with webpack-dev-server to test projects locally
- Enable hot module reloading to view changes instantly
Getting a WebXR-ready Browser
- Use an evergreen browser like Firefox or Chrome with WebXR support
- Install the WebXR emulator extensions for testing without a VR headset
- Enable experimental WebXR features on Chrome to access the latest APIs.
2. Integrating WebXR with Three.js
With the development environment set up, we can now integrate WebXR capabilities into our Three.js projects. Here is an overview of bringing in WebXR support and interactivity into a Three.js scene:
WebXR support in Three.js and available APIs
- Three.js has integrated classes for enabling AR/VR rendering
- Scenes can be easily rendered in stereo using a WebXR camera
- Has controls to start/end an XR session and render each frame.
Enabling WebXR features in your Three.js projects
Check for WebXR compatibility on the user’s browser
- Request an XR session using the “
navigator.xr.requestSession()
- Create a
WebXRManager
instance to handle rendering - Pass a
WebXRCamera
to the scene for stereo views
3. Building a Simple WebXR Application
Now let’s put our WebXR and Three.js knowledge into practice by planning, designing, and developing a simple immersive experience from start to finish.
Conceptualising the WebXR Application
For our project, we’ll create an underwater ocean scene that users can freely explore using VR headsets and motion controllers.
- Underwater Environment
Design a rich underwater environment filled with fish, swaying plants, coral reefs, and ocean floor cave systems. - Sunbeam Effects
Add visual interest with rays of sunlight beaming down through the water. Animate the light rays drifting gently. - Sea Creature Interactivity
Allow users to interact with fish and ocean creatures using their hand controller models. - Gaze-Based Navigation
Implement a gaze-based navigation system for wandering through the environment guided by headset pose.
Designing 3D Models and Assets
With ideas in place, we start bringing our world to life by modelling out key environmental assets and animated characters using Blender:
- Underwater Terrain and Caves
Carefully sculpt out the ocean floor with coral ridges, seaweed-dense kelp forests, and winding cave passageways. - Animated Tropical Fish
Model a diverse variety of colorful fish with bobbing fins and animated swimming actions. - Swaying Flora
Use vertex animation techniques to make lush plant life react to underwater currents with graceful sways. - Light Ray Effects
Utilise volumetric and shader tricks to cast visual sunbeams through murky waters.
Export all models, textures, and animations to optimised, web-ready GlTF format.
Implementing user interactions and navigation within the WebXR environment
With assets ready, we import them into our Three.js scene and use WebXR capabilities to craft key interactions:
- Controller Models
Render detailed models of the user’s hands holding motion controllers using input sources. - Touch Interactions
Detect trigger presses on controllers to select fish and have them follow while held. - Gaze Highlighting
Raycast fish based on the VR headset orientation to highlights targets for selection. - Headset-Based Steering
Transform the camera rig based on head pose rotations allowing users to peer around and swim through the underwater sandboxes.
The result is an immersive undersea environment brought to life that VR users can freely discover!
4. Testing and Debugging
Before releasing your WebXR experience, rigorous testing across devices and browsers is a must to catch issues.
Testing WebXR application on different devices and browsers
- Test VR mode on both mobile AR and tethered VR headsets
Use emulator extensions to test full experience without headsets
Verify experience works properly in AR mode on phones
Debugging common issues and errors in WebXR development
- Inspect browser console logs for errors with XR session requests
Check controller inputs are mapped correctly on all devices
Troubleshoot rendering issues related to stereo views
Utilising developer tools and browser extensions for troubleshooting
- Browser dev tools to monitor performance and OpenGL errors
WebXR extensions that provide specialised debugging data
Three.js inspector extension to visualise scene hierarchy
5. Optimization and Performance
To ensure buttery smooth 60fps framerates critical for immersion, we need to optimise our WebXR apps by:
Optimising 3D Assets
- Use optimised low-poly models instead of high-poly raw exports
- Simplify materials and textures to only essential properties
- Batch models into single geometries where possible
Efficient Rendering Techniques
- Implement occlusion culling to skip hidden objects
- Use static-baked lighting instead of expensive dynamic lights
- Render to res offscreen buffers first
Reducing Motion-to-Photon Latency
- Disable v-sync and reduce anti-aliasing for faster frame rates
- Prioritize time-critical calculations before non-essential ones
- Prevent accidental duped draw calls using layer
Test on target hardware and iterate to deliver smooth 90fps experiences!
6. Deployment and Distribution
Once thoroughly tested and optimised, it’s time to share your WebXR creation with the world!
Packaging your WebXR application for deployment
- Use Webpack to bundle source code and assets into production builds
- Minify and tree shake to optimise file size footprint
- Add app icons and metadata for app-like installable experience
Hosting considerations and options for sharing your projects online
- Host optimised build on services like Vercel, Netlify, or AWS
- Submit to WebXR app libraries like IMMERSED
- Share on social channels with preview images/video
Promoting your WebXR application through social media and online communities
- Post on WebXR developer communities like Reddit
- Show behind the scenes sneak peeks on Twitter or YouTube
- Attend virtual or in-person developer conferences
Summing it up
We just covered everything you need – from environment setup, to coding with Three.js, all the way to troubleshooting and distribution tips – to develop amazing WebXR apps from scratch. Lowering the barriers to immersive development, WebXR promises to make virtual worlds on the open web the next explosive trend in technology.
So what are you waiting for? It’s a great time to skill up on WebXR and shape how the next generation will learn, play, and connect online!
If you are looking to build a custom WebXR experience but don’t have the specialised development resources in-house, consider utilising an experienced WebXR agency like Threejsdevelopers.com. Taking on all the difficulties of creating, evaluating and maintenance, threejsdevelopers.com permits businesses to zero in on their central business targets instead of building tech expertise.
Exploring 3D Objects
Mastering Three.js for game development is an ongoing journey of continuous learning and experimentation. These tips and tricks serve as a roadmap, empowering developers to create captivating 3D games that redefine web-based gaming experiences. As the landscape evolves, commitment to ongoing learning becomes crucial for staying at the forefront of innovative game development. So, dive into the code, experiment with new techniques, and let your creativity flourish in the dynamic world of Three.js. Happy coding!